?print?
-
int?msgget(key_t,?key,?int?msgflg);??
?print?
-
int?msgsend(int?msgid,?const?void?*msg_ptr,?size_t?msg_sz,?int?msgflg);??
?print?
-
struct?my_message{??
-
????long?int?message_type;??
-
????/*?The?data?you?wish?to?transfer*/??
-
};??
?print?
-
int?msgrcv(int?msgid,?void?*msg_ptr,?size_t?msg_st,?long?int?msgtype,?int?msgflg);??
?print?
-
int?msgctl(int?msgid,?int?command,?struct?msgid_ds?*buf);??
?print?
-
struct?msgid_ds??
-
{??
-
????uid_t?shm_perm.uid;??
-
????uid_t?shm_perm.gid;??
-
????mode_t?shm_perm.mode;??
-
};??
?print?
-
#include?
?? -
#include?
?? -
#include?
?? -
#include?
?? -
#include?
?? -
#include?
?? -
struct?msg_st??
-
{??
-
????long?int?msg_type;??
-
????char?text[BUFSIZ];??
-
};??
-
int?main()??
-
{??
-
????int?running?=?1;??
-
????int?msgid?=?-1;??
-
????struct?msg_st?data;??
-
????long?int?msgtype?=?0;?//注意1??
-
????//建立消息隊列??
-
????msgid?=?msgget((key_t)1234,?0666?|?IPC_CREAT);??
-
????if(msgid?==?-1)??
-
????{??
-
????????fprintf(stderr,?“msgget?failed?with?error:?%dn”,?errno);??
-
????????exit(EXIT_FAILURE);??
-
????}??
-
????//從隊列中獲取消息,直到遇到end消息為止??
-
????while(running)??
-
????{??
-
????????if(msgrcv(msgid,?(void*)&data,?BUFSIZ,?msgtype,?0)?==?-1)??
-
????????{??
-
????????????fprintf(stderr,?“msgrcv?failed?with?errno:?%dn”,?errno);??
-
????????????exit(EXIT_FAILURE);??
-
????????}??
-
????????printf(“You?wrote:?%sn”,data.text);??
-
????????//遇到end結束??
-
????????if(strncmp(data.text,?“end”,?3)?==?0)??
-
????????????running?=?0;??
-
????}??
-
????//刪除消息隊列??
-
????if(msgctl(msgid,?IPC_RMID,?0)?==?-1)??
-
????{??
-
????????fprintf(stderr,?“msgctl(IPC_RMID)?failedn”);??
-
????????exit(EXIT_FAILURE);??
-
????}??
-
????exit(EXIT_SUCCESS);??
-
}??
?print?
-
#include?
?? -
#include?
?? -
#include?
?? -
#include?
?? -
#include?
?? -
#include?
?? -
#define?MAX_TEXT?512??
-
struct?msg_st??
-
{??
-
????long?int?msg_type;??
-
????char?text[MAX_TEXT];??
-
};??
-
int?main()??
-
{??
-
????int?running?=?1;??
-
????struct?msg_st?data;??
-
????char?buffer[BUFSIZ];??
-
????int?msgid?=?-1;??
-
????//建立消息隊列??
-
????msgid?=?msgget((key_t)1234,?0666?|?IPC_CREAT);??
-
????if(msgid?==?-1)??
-
????{??
-
????????fprintf(stderr,?“msgget?failed?with?error:?%dn”,?errno);??
-
????????exit(EXIT_FAILURE);??
-
????}??
-
????//向消息隊列中寫消息,直到寫入end??
-
????while(running)??
-
????{??
-
????????//輸入數據??
-
????????printf(“Enter?some?text:?“);??
-
????????fgets(buffer,?BUFSIZ,?stdin);??
-
????????data.msg_type?=?1;????//注意2??
-
????????strcpy(data.text,?buffer);??
-
????????//向隊列發送數據??
-
????????if(msgsnd(msgid,?(void*)&data,?MAX_TEXT,?0)?==?-1)??
-
????????{??
-
????????????fprintf(stderr,?“msgsnd?failedn”);??
-
????????????exit(EXIT_FAILURE);??
-
????????}??
-
????????//輸入end結束輸入??
-
????????if(strncmp(buffer,?“end”,?3)?==?0)??
-
????????????running?=?0;??
-
????????sleep(1);??
-
????}??
-
????exit(EXIT_SUCCESS);??
-
}??

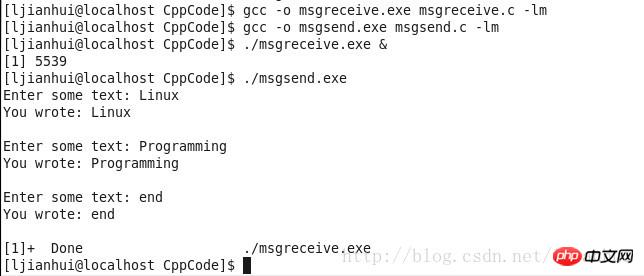
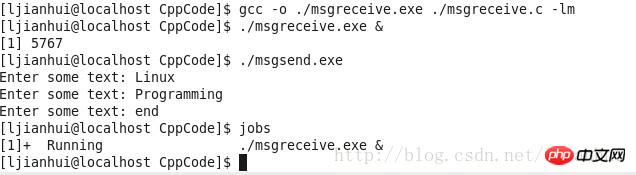